Python tip: Use for loops correctly
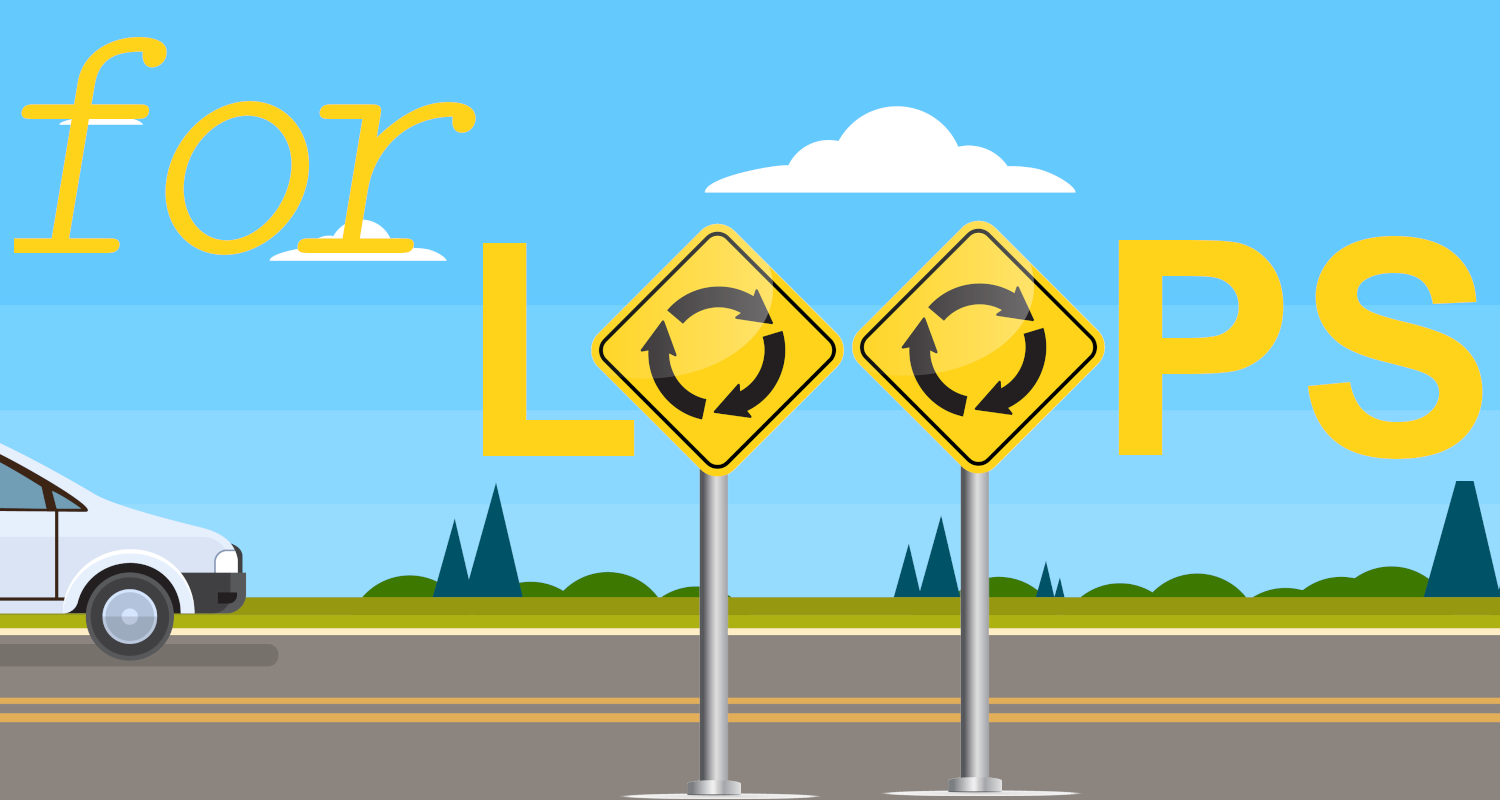
If you come from another language, like C, you may write a loop like this:
names = 'Erik', 'Alex', 'Saskia', 'Ahmed'
i = 0
while i < len(names):
print(names[i])
i = i + 1
Erik
Alex
Saskia
Ahmed
Or perhaps you have discovered Python’s for loop so you use that to make i equal to 0, 1, 2 and then 3:
names = 'Erik', 'Alex', 'Saskia', 'Ahmed'
for i in range(len(names)):
print(names[i])
Python has something called ‘iterators’, things you can ‘iterate’ (loop) over. For instance lists, tuples, sets and strings.
(Technically these are iterables which can return an iterator, but that is far too much detail at this point).
The for loop asks the list for the first element, then the next element, etc. until the whole list has been used.
We use it like this:
names = 'Erik', 'Alex', 'Saskia', 'Ahmed'
for name in names:
print(name)
The output is exactly the same.
This expresses what is happening much clearer. It almost reads like English: “For each name, print it”