Python tip: 1. 2. 3. Enumerate
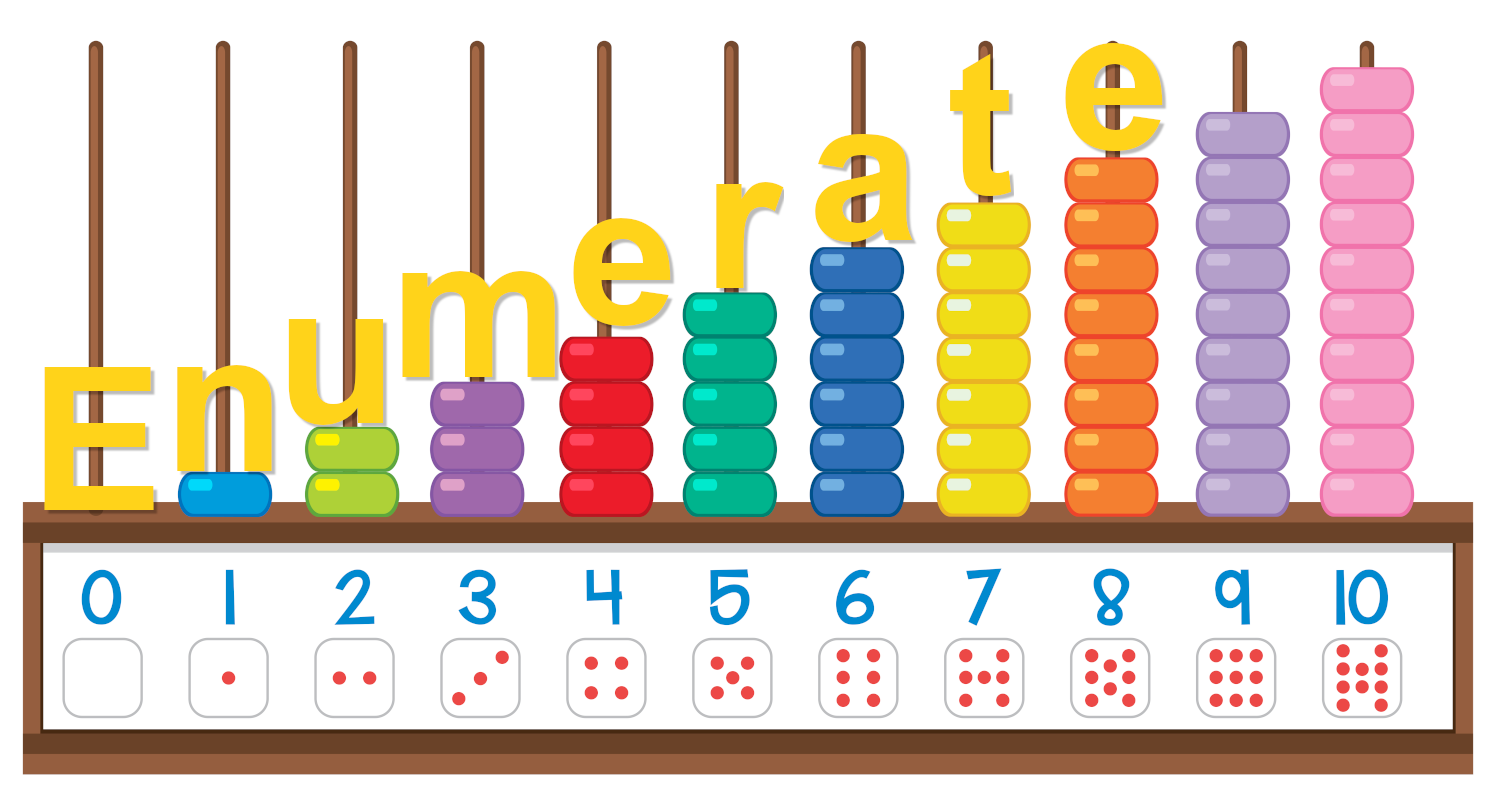
Say you want to print a numbered list. For instance:
- Apples
- Bananas
- Oranges
- Pineapple
How would you do this?
Maybe like this:
fruit = 'Apples', 'Bananas', 'Oranges', 'Pineapple'
index = 1
for one_fruit in fruit:
print(index, '.', one_fruit)
index += 1
1 . Apples
2 . Bananas
3 . Oranges
4 . Pineapple
(The output has a space after each number, which doesn’t look right. This article is about enumeration. We’ll keep this simple for now and tidy it up at the very end)
Or you code it like this:
for i in range(len(fruit)):
print(i + 1, '.', fruit[i])
That is one line shorter with the same output. However, it is not very readable. It tells Python how to get the job done, in fine detail. We should let Python do the thinking instead.
Using the enumerate function we can simplify this:
for i, one_fruit in enumerate(fruit):
print(i, '.', one_fruit)
0 . Apples
1 . Bananas
2 . Oranges
3 . Pineapple
On each iteration (each time we go through the loop) enumerate returns the next index and the next element from our list.
However, the numbers now start with 0 (zero). We wanted it to start with 1 (one). This is how we can fix it:
for i, one_fruit in enumerate(fruit, 1):
print(i, '.', one_fruit)
1 . Apples
2 . Bananas
3 . Oranges
4 . Pineapple
And finally, let’s just tidy up the output using an f-string. I may say more about this in a later article. Here is the final code:
for i, one_fruit in enumerate(fruit, 1):
print(f'{i}. {one_fruit}')
1. Apples
2. Bananas
3. Oranges
4. Pineapple