A simple GUI (graphical user interface)
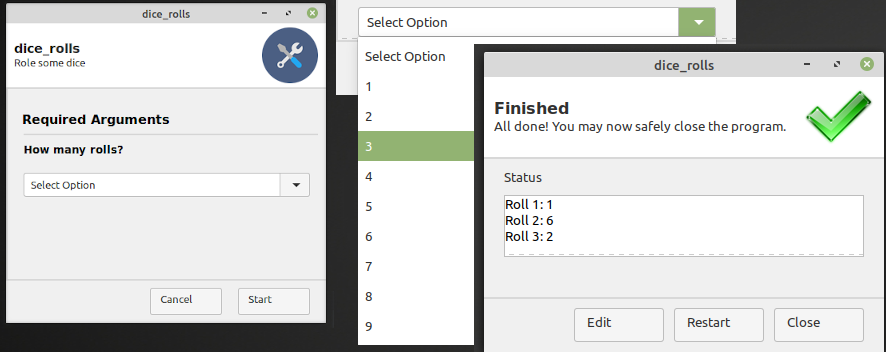
Python is an easy-to-learn yet powerful language, and comes with a wealth of libraries.
It is often used to create simple scripts. You run these on the command line, giving it any parameters it needs. It can be tricky to remember the parameters. Many people prefer to use a graphical user interface (GUI, pronounced ‘gooey’) instead. This typically contains a form which asks for the required information.
A single library, and a few lines of code, turn the manual parameter entry into a graphical user interface (GUI, pronounced ‘gooey’). The app shown above took just 10 lines of code, using Gooey.
Gooey promises to “Turn (almost) any Python command line program into a full GUI application with one line”.
Before installing Gooey, to keep your Python version(s) clean, you may want to set up a virtual environment first.
To install Gooey, use ‘pip install gooey’.
Here is the annotated code. You can find the raw code at the GitHub repository
1. import random 2. import gooey Load the necessary libraries. ‘random’ to create some random numbers (roll the dice). And ‘gooey’ to create the GUI
3. @gooey.Gooey(default_size=(300, 300), show_success_modal=False) When running this function, run it using Gooey. Set the window to 300 x 300. Don’t show a modal success window when done, just show the results
4. def main(): Start defining the ‘main’ function
5. parser = gooey.GooeyParser(description=’Role some dice’) Create an argument parser. Instead of the command line ‘argparse’ parser from Python’s standard library we will be using Gooey’s GUI equivalent
6. parser.add_argument(‘number_of_rolls’, type=int, metavar=’How many rolls?’, choices=range(1, 10)) Tell the argument parser that the first (and only) parameter is an integer, with a value from 1 to 10 (exclusive, i.e. stop just before 10)
7. args = parser.parse_args() Show the GUI to get the argument(s)
8. for i in range(args.number_of_rolls): The value entered (in the input box) or chosen (from the dropdown) is returned in args_number_of_rolls. Execute the following line that many times
9. print(f’Roll {i + 1}: {random.randint(1, 6)}’) Print a sequence number (‘Roll 1’, ‘Roll 2’, etc), followed by a random number from 1 to 6 (inclusive, so one of 1, 2, 3, 4, 5 or 6)
10. main() Call the main() function, start the program