Python tip: Assignment operators
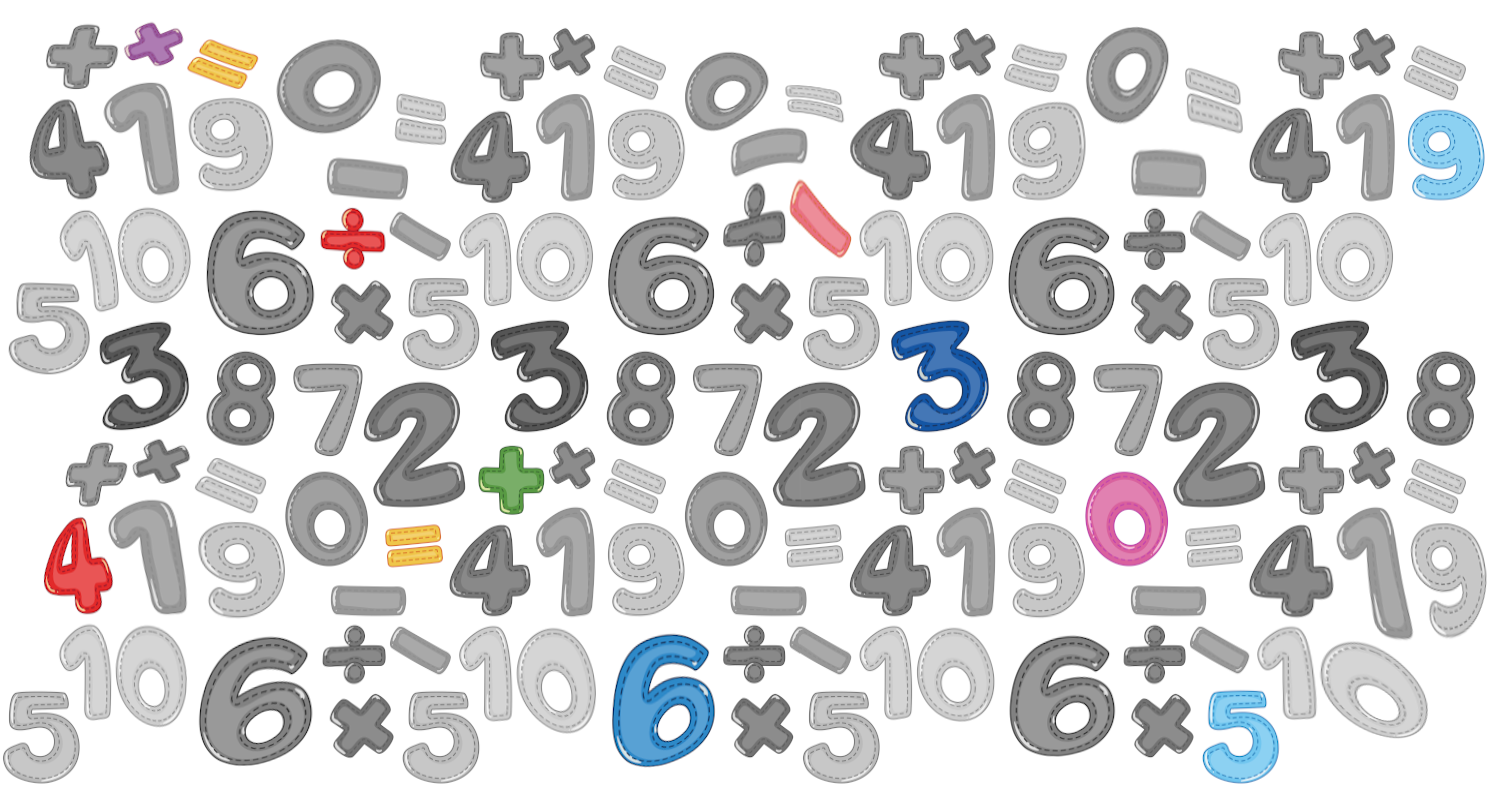
How do you increase the value of a variable?
number = 10
number = number + 5
print(number)
15
Here are a few more operations:
number = 10
number = number - 5
number = number * 2
number = number / 3
number = number ** 2
number = number // 4
print(number)
2.0
If you don’t know some of these operators (e.g. ** or //) check out the Python documentation.
Notice how we use the variable name twice on each line. We can shorten this:
number = 10
number += 5
print(number)
15
” += ” is an “assignment operator”. It is both a operator (+) and an assignment (=)
You can do the same for the other operators:
number = 10
number -= 5
number *= 2
number /= 3
number **= 2
number //= 4
print(number)
2.0
With lists – advanced gotcha
Here is some more advanced information. If you are new to Python just ignore this. You may never need to know this. I’m mostly adding this for completeness and to show off ;-).
If you start using assignment operators all the time there is a small chance that this will catch you out some day.
Assignment operators make your code more expressive and may prevent errors. Most of the time you can think of the following two lines doing the same thing:
a = a + b
a += b
This works for integers and floats, but also for tuples and lists:
names = ['Fred', 'Annelies']
names += ['Alex']
print(names)
['Fred', 'Annelies', 'Alex']
However, for lists, assignment operators are not exactly the same as normal operators.
# Version A
names = ['Fred', 'Annelies']
names_copy = names
names = names + ['Alex']
print(names_copy)
['Fred', 'Annelies']
# Version B
names = ['Fred', 'Annelies']
names_copy = names
names += ['Alex']
print(names_copy)
['Fred', 'Annelies', 'Alex']
Versions A and B are almost exactly the same. The normal operator in Version A is replaced by an assignment operator in version B, but that is all. Yet they produce a different result.
In Version A the addition in “names = names + [‘Alex’] ” creates a new list, but leaves the old list unchanged. The old list is still referenced by the name_copy variable. When we print it we get the old list with Fred and Annelies.
In Version B “names *= [‘Alex’] ” is an in-place operation. It changes the list itself instead of creating a new list. Because no new list was created there is still only one list. The names and names_copy variable both reference this list. A new value was added to this. Whether we print names or names_copy we get all three names including Alex.
# Version C
names = ['Fred', 'Annelies']
names_copy = names
names.extend(['Alex'])
print(names_copy)
['Fred', 'Annelies', 'Alex']
According to the official documentation, for a mutable sequence such as a list, “s.extend(t)” or “s += t” both do the same thing: “extends s with the contents of t”. As you can see, Version B and Version C give the same results.